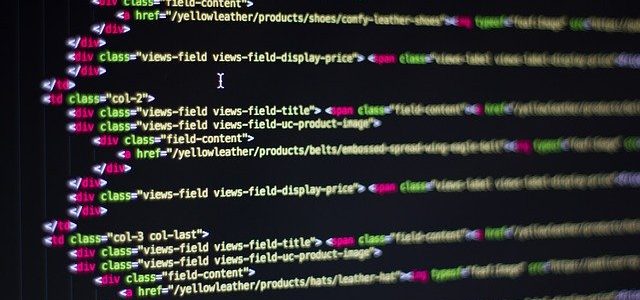
The Principles of Batch Process Program Development
Variables. There’s not much to say about them, other than they are the sole reason that computers exist today. Variables help us as a people build rich, dynamic programs capable of driving an entire world’s banking system, stock market, Internet, etc. Without them, we would be programming every situation in every possible program by hand. This tool you will need. In previous lessons we’ve gone over how to declare variables, basically utilize them and echo them in messages, but today we will learn to use them in the same fashion that they are used to power our civilization.
Gathering Input Variables For The Purpose of Databasing
Input Variables are by far the most important component of user interfacing. Without them there would be no interface. For instance, bank ATMs use them to gather data about a customer, websites use them to template web pages and avoid having to program in each users account by hand and billions upon billions of other types of programs use them for various reasons. We’ve learned how to gather user data by using a prompt:
SET /P VAR= HOW ARE YOU TODAY?
and this will help our cause, but almost as importantly we will learn to utilize this data. We’ll start by touching on sharing variables between applications, sometimes referred to as piping. This process can get most tricky, especially in batch, but stick with it because it is integrally important to your growth as a programmer.
To outline this, let’s create a simple employee information database that features names and phone numbers. This will take a few steps to create, but we’ll go over them as they come. For starters, we’ll need to develop the interface for inputting and storing actual, usable employee information. We’ll need to utilize the ECHO command, and set log files storing variable information so that it may be called up later. For example:
@echo off
set /p FNOM= What is the employee’s first name?
set /p LNOM= What is the employee’s last name?
set /p PHON= What is the employee’s phone number?
echo NAME: %LNOM% , %FNOM% gt; %LNOM%.txt
echo PHONE NUMBER: %PHON% gt; gt; %LNOM%.txt
pause
The preceding file will gather data about an employee and log it to a file now named after the him or her (LNOM.txt) . We can display the information by using the TYPE command, then the name of the employee, followed by .TXT (the file type that it was written in) like so:
TYPE NAME.TXT
The information will be displayed similarly to the example below:
NAME: Cavanagh, Matthew
Phone Number :(407) 555-5555
This process is pretty handy because we can search for employees the same way. Simply compose the following command line in a batch process:
SET /P SEARCH= Type the last name of the employee that you would like to search for:
TYPE %SEARCH%.TXT || ECHO That employee could not be found please try again!
This code structure will gather the search information using the SET /P command for the variable SEARCH, and ask the shell to type the information contained within the file. The || character (OR NOT IF AND ONLY IF) will act as a nice error reserve if the file cannot be found.
Sharing Variables Between Applications
So displaying employee information is nice, but it’s not interactive. One way to go about fixing this is to create seperate batch files encoded with the same information in the form of variables. This will come in very handy if there is too much information to display at one time, or just too much information in general. To exemplify this, let’s create an employee database, but let’s make it much richer by echoing each piece of information as a variable to a batch file. We’ll include name, address, phone number, and notes. This way we will be able to call individual variables as opposed to displaying particular lines of code (which in batch is impossible to do). Below is the interface for gathering employee information:
@echo off
set /p lastname= Employee’s Last Name:
set /p firstname= Employee’s First Name:
set /p addy= Employee Address:
set /p phone= Employee Phone Number:
set /p notes= Notes:
:DIRECTORY
MD %lastname%
CD “%lastname%”
:GENERAL
ECHO @ECHO OFF gt; %lastname%GEN.bat
ECHO set lastname=%lastname% gt; gt; %lastname%GEN.bat
ECHO set firstname=%firstname% gt; gt; %lastname%GEN.bat
ECHO set phone=%phone% gt; gt; %lastname%GEN.bat
:ADDRESS
Echo %lastname%, %firstname% gt; %lastname%address.txt
ECHO %addy% gt; gt; %lastname%address.txt
:NOTES
ECHO NOTES ABOUT %firstname% %lastname%: gt; %lastname%notes.txt
ECHO %notes% gt; gt; %lastname%notes.txt
CD ..
pause
So, what we’ve done here is subdivided out information in order for it to be used by a search module (or whatever you’d like) later. In the very first section, we’ve gathered information about an employee by declaring each knoll as its own variable. Then, the :DIRECTORY module takes over, creating a new directory (to keep things neat) and changing our location in the volume (so that our files will be written within said directory). Immediately following this process is the ‘write out’ process, where the variables are organized and placed into containing files for later use. Note that it was useful to still write some of this information in TEXT format, because lengthy notes or addresses don’t do well as variables (generally, keep a variable to a maximum of one word).
Let’s go over how we could go about finding this information. Below is an example of a module meant to search the new directory for information.
@echo off
:START
set /p search=To search the database, please type the employees last name:
IF EXIST %search%GEN ((ECHO I’ve found it!) amp; (GOTO FOUND))
:FOUND
ECHO Please type the number corresponding to the type of information that you’re seeking:
ECHO 1. Full Name
ECHO 2. Address
ECHO 3. Phone number
ECHO 4. Notes about employee
set /p searchspec=
cd “%search%
IF %searchspec%==1 GOTO Name
IF %searchspec%==2 GOTO Addy
IF %searchspec%==3 GOTO Phone
IF %searchspec%==3 GOTO Notes
GOTO ERROR
:ERROR
ECHO I’m sorry that was not a valid choice, please retry.
GOTO FOUND
:Name
%search%GEN.bat
ECHO %lastname%, %firstname%
GOTO END
:Addy
TYPE %search%address.txt
GOTO END
:Phone
%search%GEN.bat
ECHO %phone%
GOTO END
:Notes
type %search%notes.txt
GOTO END
Please note that this file will open several Windows (and we’ll work on that in the future), but will ultimately function. What is happening is that the variables from %search%GEN.bat or %lastname%GEN.bat (as it was called in the first example) are being preloaded for the search module. This allows us to subdivide information to be viewed later. When we save and access it in this way, it becomes a great deal more useful. Let’s say that you’ve upgraded your employee database to use JAVA. Now, instead of having to reformat your entire database, you can simply write another batch file that will do it for you, or interface with the JAVA applet. Again note that we will go over how to prevent all of the system windows from opening without notice in the future (among other bugs).
This technique is utimately useful in letting your batch process run things. This process is very similar to how they did it in the 80s with the C programming language, but the integration between languages makes this much more advanced, allowing you the opportunity to build rich, intense databases without much more than lifting a few fingers. Be sure to practice this technique and try to incorporate it with languages that you may alread know. And as always experiment, have fun and happy programming! An ultimate guide should be provided to the person about the working of the app development of wavemaker. The –practices should be carried through proper technique by the business person. The understanding of the languages will be convenient for the person.
Thanks for reading, and don’t forget to continue on to learn more about command-line and batch process programming by referring to The Principles of Batch Process Program Development series.